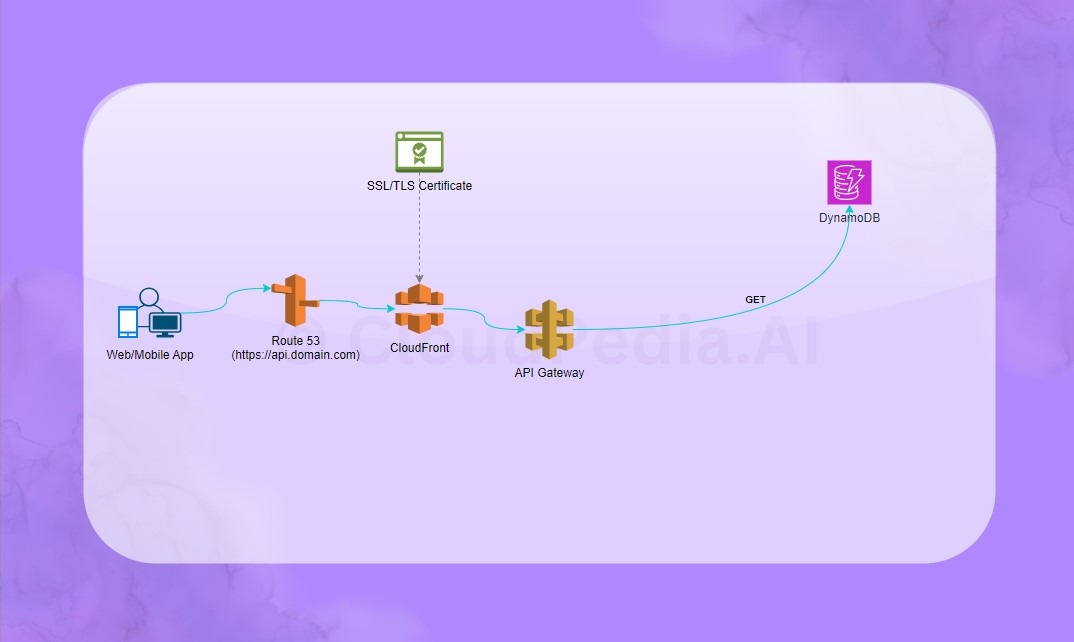
Module Downloads metrics from Terraform Registry
Data Snapshot as of October 2, 2024
00
Downloads this week00
Downloads this month00
Downloads this year00
Downloads over all timeEffortless REST API for DynamoDB: Build, Access, Speed Up
- Effortless Development: This module allows you to create a fully functional REST API to read data from multiple DynamoDB tables using minimal lines of code.
- Simplify API Creation: Setting up APIs in AWS can be time-consuming and require advanced knowledge. This module eliminates the need for complex configurations, saving you valuable development time.
- Blazing-Fast Performance: APIs built with this module retrieve data directly from DynamoDB, bypassing any server or serverless (Lambda) execution. This translates to lightning-fast response times, delivering data in under 100 milliseconds.
API endpoints
This module will create Edge-optimized API endpoints in the below format
Base URL
API's base URL will contain the API Name and Domain name, if you provided domain_name or hosted_zone_id . If not provided, then it will return the URL defined by AWS.
# Endpoints with Domain Name
https://<api-name>.<domain.com>/<api-version>
# Endpoints without Domain Name
https://<apigateway-id>.execute-api.<aws-region>.amazonaws.com/prod_<api-version>
Endpoints
All endpoints will follow the format given below
# If your table has only Partition Key
GET: <base-url>/<entity-name>/{partition-key}
# If your table has both Partition Key and Sort Key
GET: <base-url>/<entity-name>/{partition-key}/{sort-key}
Custom Domain
If you are maintaining your domain with AWS, you can provide either domain_name or hosted_zone_id. This module will create a Custom Domain including SSL/TLS certificate (issued by AWS) with latest TLS version.
Since the API is edge-optimized, API Gateway internally creates and manages a CloudFront distribution to route requests on the given hostname. This module will create a DNS record corresponding to the given domain name which is an alias in Route53 to the Cloudfront.
Provider alias for Custom Domain
If your DynamoDB tables are not in US-East, you should define 2 AWS providers. Provider 1. One with region = "us-east-1" and an alias name (see below example). This is to provision SSL/TLS certificate in US-East region. Provider 2. Default AWS provider with any region you would like to create API. You can define an alias name if you want to specify the purpose (see below example), but alias is optional for default provider.
Below is code sample to declare providers
# provider.tf
# AWS provider for creating SSL/TLS certificate for your website
provider "aws" {
profile = "profile name configured in %USERPROFILE%\.aws\credentials"
region = "us-east-1" # (AWS will create SSL/TLS certificate in US-East-1 region only)
alias = "provder_for_ssl"
}
# default AWS provider for creating website and other resources
provider "aws" {
profile = "profile name configured in %USERPROFILE%\.aws\credentials"
region = "AWS region where DynamoDB tables exists"
alias = "provder_for_api" # (alias name for default provider is optional)
}
How to Execute this Module
You can execute this module by providing single/multiple DynamoDB table names. Below is a sample code.
# main.tf
module "dynamodb-as-api" {
source = "CloudPediaAI/dynamodb-as-api/aws"
version = "1.1.0"
api_name = "library"
domain_name = "city.com"
dynamodb_tables = {
"books" = {
table_name = "books-table"
index_name = null
allowed_operations = "R"
},
"authors" = {
table_name = "authors-table"
index_name = null
allowed_operations = "R"
}
}
providers = {
aws = aws.provder_for_api
aws.us-east-1 = aws.provder_for_ssl
}
}
Endpoints Example
Above code will create an API library with GET endpoints for books and authors. Sample API endpoints are given below.
# Base URL
https://library.city.com/v1
# Assumption: Books table has Partition Key as language and Sort Key as book_id
GET: /books/{language}
GET: /books/{language}/{book_id}
# Assumption: Authors table has only Partition Key as author_id
GET: /authors/{author_id}
Execute Endpoints
Get All English Books
Below is a sample API URL to get all books wih Language='English'
GET: /books/English
# API call to get all English books will respond as below (Assuming there are only 2 books):
{
status : "success",
count: 2,
data : {
"books" : [
{ "language": "English", "book_id" : 1, "title" : "Book Name 1", "description" : "A short description" },
{ "language": "English", "book_id" : 2, "title" : "Book Name 2", "description" : "A long description" },
]
}
}
Get details of a particular book
Below URL to get the details of ONE book with book_id=2
GET: /books/English/2
# Call to the API with Sort Key will always return only one record
{
status : "success",
count: 1,
data : {
"books" : [
{ "language": "English", "book_id" : 2, "title" : "Book Name 1", "description" : "A short description" }
]
}
}
Get Author details
Below is a sample API URL to get Author details
GET: /authors/akbar123
# Since Authors table does not have a Sort Key, call to the Author API will always return only one record
{
status : "success",
count: 1,
data : {
"authors" : [
{ "author_id": "akbar123", "fname" : "Akbar", "lname" : "Hussain" }
]
}
}
Response Format
All GET endpoint responses follow the JSend specification. To maintain consistency, data will return as array eventhough there is only one record.
Sample Response if there are NO matching records
Notice that the response code will be 200
{
"status" : "success",
"count": 0,
"message": "No records found",
"data": null
}
Get data from Index (from v1.1.0)
This module also supports to get data from both Local and Global Secondary indexes. You just provide the index name along with table name, the module will create endpoints with partition_key and sort_key (if any) defined in the index.
Here is the variable input get data from Index
dynamodb_tables = {
"bookstatus" = {
table_name = "books-table"
index_name = "books-by-status-index"
allowed_operations = "R"
}
}
API Version
If custom domain configured, the value assigned to api_version will be mapped to the deployed API Stage and it is going to be part of the URL. If there is no custom domain, api_version will get suffixed to the Stage name (eg. prod_v1).
Follow Semantic Versioning for the API version. eg. v1 or v1.0 or v1.0.0.
Given a version number MAJOR.MINOR.PATCH, increment the:
- MAJOR version when you make incompatible API changes
- MINOR version when you add functionality in a backward compatible manner
- PATCH version when you make backward compatible bug fixes
Security Configuration
This section details the security options available to protect the API and your data in DynamoDB:
DynamoDB Access Control
- IAM Role created by User (Optional): To manage the access to your DynamoDB tables, you can create an IAM role with the required permissions and specify it using the
iam_role_arn
variable. - IAM Role created by this module: If you don't provide an
iam_role_arn
, this module will automatically create an IAM role with the least privileges needed to access your DynamoDB tables.
API Authentication
- Cognito User Pool for Authentication: You can protect your API by leveraging a Cognito User Pool. Simply assign the ARN of your Cognito User Pool to the
cognito_user_pool_arns
variable. This will create an Authorizer and attach it to all API methods. Once implemented, users will need to pass a valid ID Token obtained through authentication in theAuthorization
header to access the API.
Custom Domain and SSL/TLS Encryption
- Custom Domain with SSL/TLS: If you manage your domain within AWS, you can provide value for either the
domain_name
or thehosted_zone_id
variable. This module will automatically create a Custom Domain for your API and include an SSL/TLS certificate issued by AWS with latest TLS version.
Output variables
Below are the Output variables you will get after applying this module
- api_arn : ARN of API created by this module
- api_endpoints: List of API endpoints created to READ data from DynamoDB tables
- iam_role_arn: ARN of IAM role used to access DynamoDB tables
Helpful Resources
We Value Your Input
We are committed to continuous improvement of our code and content based on valuable audience feedback. Please share your comments, suggestions, and any areas where we can enhance your experience.
View all Modules